Intelligent Virtual Assistants: Translation with AI Agents
Explore how intelligent virtual assistants with AI translation capabilities are transforming multilingual communication. Learn about the technology behind AI translation agents, their real-world applications, and how they can break down language barriers in global business, healthcare, education, and more.
In today's globalized world, language barriers can hinder effective communication across borders. Imagine attending an international meeting where participants speak different languages, and you need to understand every word in real-time. Traditional solutions often involve human interpreters or clunky translation devices, but these approaches can be expensive, time-consuming, and sometimes inefficient.
Enter intelligent virtual assistants (IVAs) with AI translation capabilities—a revolutionary solution that's transforming how we communicate across languages. This article explores how these sophisticated AI systems are breaking down language barriers and creating seamless multilingual experiences.
What are Intelligent Virtual Assistants (IVAs) and AI Translation Agents?
Defining Intelligent Virtual Assistants (IVAs)
Intelligent Virtual Assistants are AI-powered automated agents designed to provide human-like support and interaction. Unlike simple chatbots, IVAs leverage advanced technologies including natural language understanding (NLU), machine learning, and knowledge bases to comprehend user intent and context, delivering more sophisticated and personalized experiences.
The Role of AI Translation Agents Within IVAs
AI translation agents are specialized IVAs that focus on breaking down language barriers by enabling real-time communication between people who speak different languages. These agents can:
- Listen to speech in one language
- Process and understand the content
- Translate it accurately to another language
- Deliver the translation as natural-sounding speech or text
The most advanced AI translation agents can handle this process bidirectionally and in real-time, creating a seamless conversation experience for all participants.
IVA vs. Chatbot vs. Voice Assistant: Key Differentiators in Translation Capabilities
Feature | Basic Chatbot | Voice Assistant | Intelligent Virtual Assistant |
---|---|---|---|
Translation accuracy | Limited, often word-for-word | Moderate, with some context understanding | High, with contextual and cultural adaptation |
Real-time capability | Usually text-only with delays | Can process simple requests in real-time | Can facilitate multi-way conversations in real-time |
Language support | Limited languages | Common languages | Extensive language support with dialect understanding |
Contextual awareness | Minimal | Basic context retention | Advanced context and conversation history tracking |
Adaptability | Fixed responses | Some personalization | Learns and adapts to user preferences and speaking styles |
How Do AI Translation Agents Work?
Let's explore the mechanics behind an AI translation agent through a real-world implementation example from VideoSDK's AI Translation Agent:
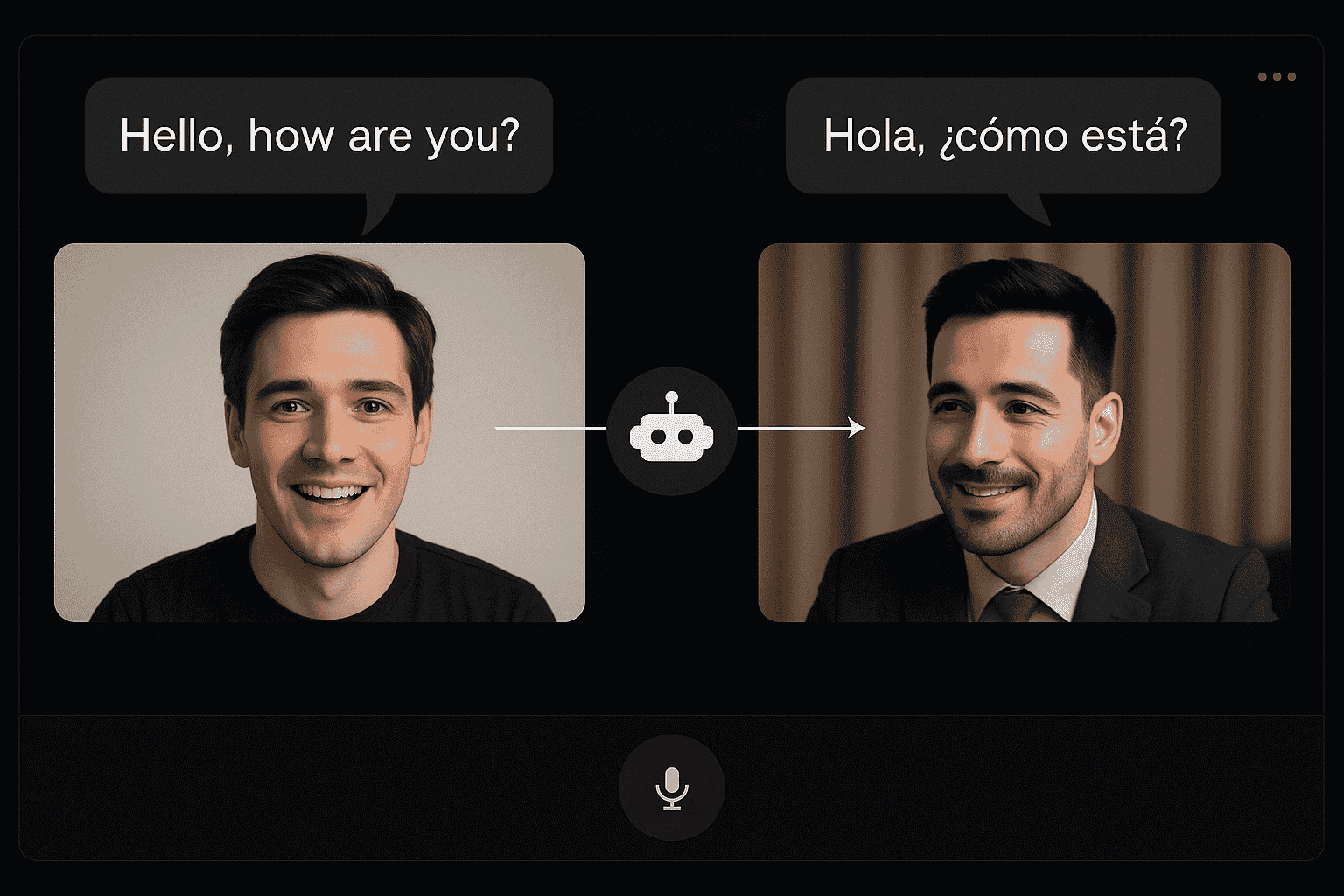
The Process of Understanding and Translating Language
The AI translation agent workflow involves several sophisticated steps:
- Audio Input Capture: The system captures audio input from participants speaking different languages.
- Speech Recognition: Converting spoken words into text using speech-to-text models.
- Language Detection: Identifying the source language automatically.
- Translation Processing: Translating the text to the target language(s).
- Text-to-Speech Conversion: Converting the translated text back to natural-sounding speech.
- Audio Output: Delivering the translated speech to the appropriate participants.
Key Technologies Powering AI Translation Agents
Looking at the code example provided, we can see several important technologies at work:
1class AIAgent:
2 def __init__(self, meeting_id: str, authToken: str, name: str):
3 # Initialize the AI agent with audio processing capabilities
4 self.audio_track = CustomAudioStreamTrack(
5 loop=self.loop,
6 handle_interruption=True
7 )
8 # Connect to OpenAI for intelligence
9 self.intelligence = OpenAIIntelligence(
10 loop=self.loop,
11 api_key=api_key,
12 base_url="api.openai.com",
13 input_audio_transcription=InputAudioTranscription(model="whisper-1"),
14 audio_track=self.audio_track
15 )
This implementation highlights the use of:
- Natural Language Understanding (NLU): Processing and understanding the meaning behind spoken words
- Machine Translation: Converting content from one language to another while preserving meaning
- Real-time Audio Processing: Capturing, processing, and generating audio with minimal latency
- WebRTC Technology: Enabling real-time communication between participants
Dynamic Instruction Setting for Contextual Translation
One particularly impressive aspect of the VideoSDK implementation is how it dynamically creates translator-specific instructions based on participant information:
1# Extract the info for each participant
2participant_ids = list(self.participants_data.keys())
3p1 = self.participants_data[participant_ids[0]]
4p2 = self.participants_data[participant_ids[1]]
5
6# Build translator-specific instructions
7translator_instructions = f"""
8 You are a real-time translator bridging a conversation between:
9 - {p1['name']} (speaks {p1['lang']})
10 - {p2['name']} (speaks {p2['lang']})
11
12 You have to listen and speak those exactly word in different language
13 eg. when {p1['lang']} is spoken then say that exact in language {p2['lang']}
14 similar when {p2['lang']} is spoken then say that exact in language {p1['lang']}
15 Keep in account who speaks what and use
16 NOTE -
17 Your job is to translate, from one language to another, don't engage in any conversation
18"""
This approach ensures that the translation is precisely tailored to the specific participants and languages involved in the conversation.
Benefits of Using IVAs with AI Translation Agents
Enhanced Communication Across Language Barriers
AI translation agents enable seamless communication between individuals who speak different languages. In business settings, this can facilitate international meetings, negotiations, and collaborations without the need for human interpreters.
Cost and Time Efficiency
Traditional translation services can be expensive and require advance booking. AI translation agents provide:
- 24/7 availability
- No per-minute billing
- No scheduling requirements
- Consistent quality across sessions
Real-time Translation Capabilities
Unlike asynchronous translation services, AI translation agents work in real-time, allowing for natural conversation flow:
1async def add_audio_listener(self, stream: Stream):
2 while True:
3 try:
4 # Continuously process audio frames in real-time
5 frame = await stream.track.recv()
6 audio_data = frame.to_ndarray()[0]
7 # Process and send to OpenAI for translation
8 await self.intelligence.send_audio_data(pcm_frame)
9 except Exception as e:
10 print("Audio processing error:", e)
11 break
This code snippet demonstrates how the system continuously processes audio frames and sends them for translation with minimal delay.
Scalability Across Multiple Languages
AI translation agents can support numerous language pairs simultaneously, making them ideal for multilingual environments. The VideoSDK implementation can dynamically handle any language pair that the underlying AI model supports.
Use Cases for AI Translation Agents
International Business Meetings
AI translation agents can facilitate seamless communication in global business meetings, allowing participants to speak in their native languages while understanding others in real-time.
Multilingual Customer Support
Companies can deploy AI translation agents to provide customer support in multiple languages without having to hire multilingual support staff.
Educational Settings
Language barriers often limit access to quality education. AI translation agents can translate lectures, discussions, and educational content in real-time, making knowledge more accessible globally.
Healthcare Communication
In healthcare settings, accurate communication is critical. AI translation agents can help healthcare providers communicate effectively with patients who speak different languages, improving care quality and reducing misunderstandings.
Travel and Tourism
Travelers can use AI translation agents to communicate with locals, navigate unfamiliar environments, and enjoy deeper cultural experiences without language constraints.
Implementing an AI Translation Agent: Technical Insights
Based on the provided code implementation, here's how an AI translation agent can be built:
1. Audio Processing Pipeline
The implementation needs robust audio processing capabilities to capture, process, and generate audio in real-time:
1class CustomAudioStreamTrack(CustomAudioTrack):
2 def __init__(self, loop, handle_interruption: Optional[bool] = True):
3 super().__init__()
4 self.loop = loop
5 self._start = None
6 self._timestamp = 0
7 self.frame_buffer = []
8 # Audio configuration
9 self.sample_rate = 24000
10 self.channels = 1
11 self.sample_width = 2
12 # More audio processing setup...
2. Real-time Communication Framework
The system needs to establish real-time communication channels between participants:
1def on_meeting_joined(self, data):
2 print("Meeting Joined - Starting OpenAI connection")
3 asyncio.create_task(self.intelligence.connect())
3. AI Integration for Translation
The core of the system is its integration with AI services for translation:
1async def connect(self):
2 # Connect to OpenAI's real-time API
3 url = f"wss://api.openai.com/v1/realtime?model=gpt-4o-realtime-preview"
4 self.ws = await self._http_session.ws_connect(
5 url=url,
6 headers={
7 "Authorization": f"Bearer {self.api_key}",
8 "OpenAI-Beta": "realtime=v1",
9 },
10 )
4. Dynamic Language Detection and Routing
The system must identify which participant is speaking which language and route translations appropriately:
1def on_participant_joined(self, participant: Participant):
2 peer_name = participant.display_name
3 native_lang = participant.meta_data["preferredLanguage"]
4 self.participants_data[participant.id] = {
5 "name": peer_name,
6 "lang": native_lang
7 }
5. Bidirectional Translation Flow
For a true conversation, the translation must flow bidirectionally:
1# Dynamically tell OpenAI to use these instructions
2asyncio.create_task(self.intelligence.update_session_instructions(translator_instructions))
Future Developments in AI Translation Agents
As AI technology continues to evolve, we can expect AI translation agents to become even more sophisticated:
Enhanced Contextual Understanding
Future AI translation agents will better understand cultural nuances, idioms, and context-specific language, producing even more natural translations.
Expanded Multimodal Capabilities
Next-generation systems will incorporate visual cues, body language, and other non-verbal communication aspects to enhance translation accuracy.
Reduced Latency
Advancements in AI processing will further reduce the delay between speech and translation, creating even more natural conversation flows.
Integration with AR/VR Environments
AI translation agents will be integrated into augmented and virtual reality environments, enabling seamless multilingual communication in immersive settings.
Conclusion
Intelligent virtual assistants with AI translation capabilities are breaking down language barriers and transforming how we communicate across linguistic boundaries. From international business meetings to healthcare settings and educational environments, these sophisticated AI systems are making multilingual communication more accessible, efficient, and natural than ever before.
The VideoSDK AI Translation Agent implementation showcases the impressive capabilities of modern AI translation systems, with real-time bidirectional translation that adapts dynamically to participant languages and conversation context. As these technologies continue to evolve, we can look forward to a world where language differences no longer limit human connection and collaboration.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ