What is WebTransport Protocol?
WebTransport is a protocol designed to enable low-latency, secure, and reliable communication over the web. It provides a more flexible alternative to traditional protocols like WebSockets or HTTP/3, supporting both unidirectional and bidirectional streams. This makes it ideal for real-time applications, such as gaming, live streaming, and interactive media, where quick data transmission is crucial. WebTransport leverages underlying technologies like QUIC to optimize performance, particularly in scenarios where minimizing delay and ensuring smooth, uninterrupted communication are essential.
WebTransport, a protocol developed by the IETF, aims to address the limitations of its predecessors by providing a more robust framework for data transmission. With its focus on minimizing latency and improving throughput, WebTransport is set to revolutionize how data is transmitted over the internet, making it an essential tool for developers building next-generation web applications.
By understanding the WebTransport protocol, developers can unlock new possibilities for creating immersive and interactive web experiences. In this article, we will guide you through the process of implementing WebTransport, provide practical code examples, and discuss its real-world applications.
Understanding WebTransport Protocol
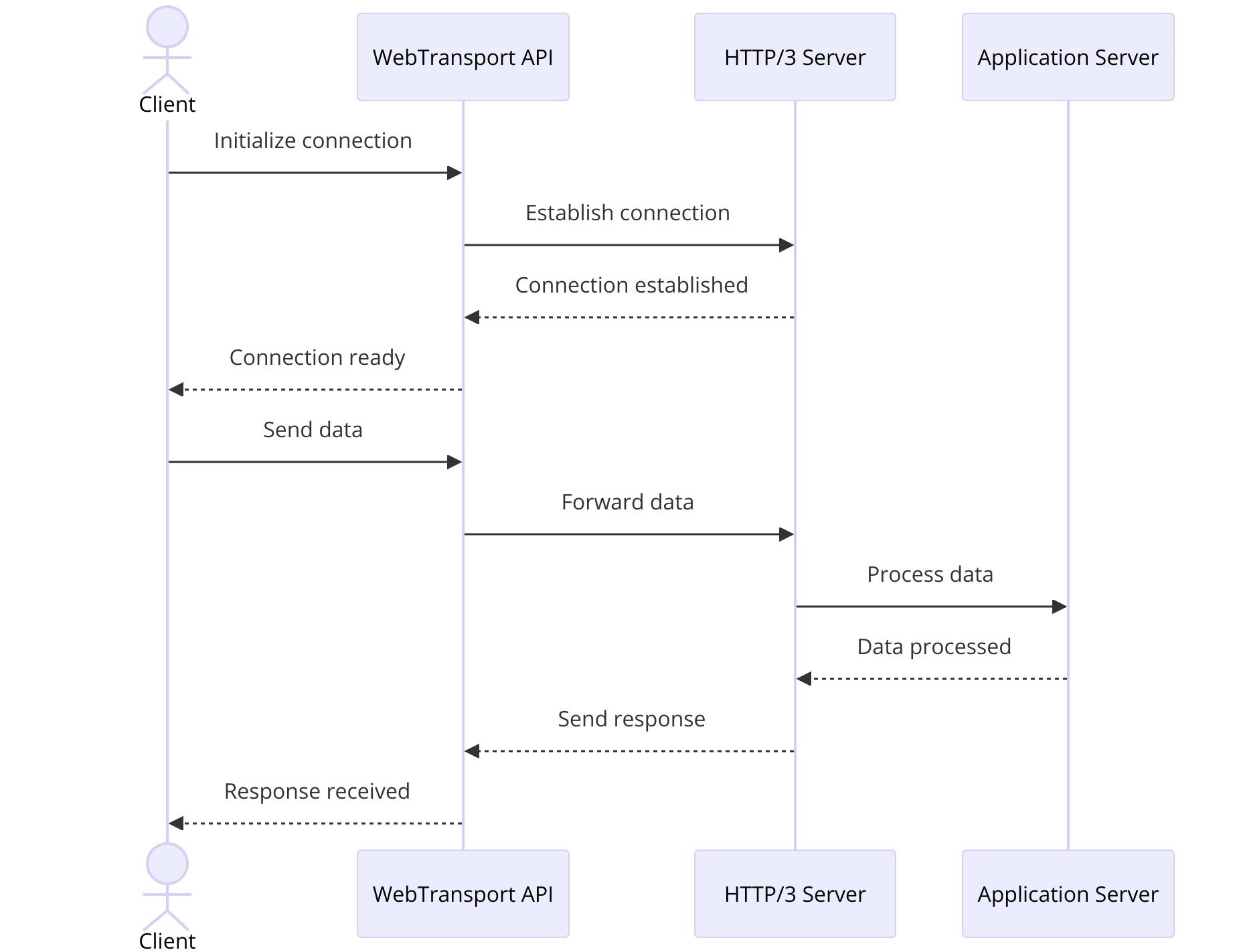
This makes it ideal for real-time applications like live streaming, online gaming, and collaborative tools. By using QUIC, WebTransport ensures improved connection reliability, faster data transfer, and better performance over varying network conditions.
Getting Started with WebTransport
Before diving into the implementation, ensure you have a modern web browser that supports WebTransport. The setup involves initializing a WebTransport connection to a specified server endpoint.
Environment Setup
- Ensure your server supports HTTP/3 and QUIC.
- Use a compatible browser (e.g., latest versions of Chrome or Firefox).
Initializing WebTransport
JavaScript
1 const transport = new WebTransport('https://example.com/echo');
2 transport.ready.then(() => {
3 console.log('Transport is ready');
4 }).catch(error => {
5 console.error('Failed to establish transport:', error);
6 });
Code Explanation
- The
WebTransport
constructor takes a URL as an argument. - The
ready
promise resolves when the connection is established, or rejects if there is an error.
Implementing WebTransport Protocol in Your Project
Establishing a Connection
After initializing, you can establish a connection and start sending or receiving data.
Sending Data
JavaScript
1 const writer = transport.datagrams.writable.getWriter();
2 writer.write(new Uint8Array([0, 1, 2, 3])).then(() => {
3 console.log('Data sent successfully');
4 }).catch(error => {
5 console.error('Failed to send data:', error);
6 });
Receiving Data
JavaScript
1 const reader = transport.datagrams.readable.getReader();
2 reader.read().then(({ value, done }) => {
3 if (done) {
4 console.log('Stream closed');
5 } else {
6 console.log('Received data:', value);
7 }
8 }).catch(error => {
9 console.error('Failed to read data:', error);
10 });
Code Explanation
writer.write()
sends data through the WebTransport connection.reader.read()
receives data from the connection.
Advanced Features and Usage of WebTransport Protocol
Stream Management
WebTransport supports bidirectional streams for more complex data transfer scenarios.
JavaScript
1 const stream = await transport.createBidirectionalStream();
2 const reader = stream.readable.getReader();
3 const writer = stream.writable.getWriter();
4
5 // Writing to stream
6 writer.write(new TextEncoder().encode('Hello, World!')).then(() => {
7 console.log('Message sent via stream');
8 });
9
10 // Reading from stream
11 reader.read().then(({ value, done }) => {
12 if (done) {
13 console.log('Stream closed');
14 } else {
15 console.log('Received stream message:', new TextDecoder().decode(value));
16 }
17 });
Handling Multiple Connections
Manage multiple connections by creating separate
WebTransport
instances for each endpoint.Security and Performance of WebTransport Protocol
Encryption
WebTransport uses QUIC, which is inherently secure due to its built-in encryption mechanisms.
Performance Optimization
- Reduce latency by minimizing the size of data packets.
- Utilize streams for large data transfers to maintain connection stability.
Common Pitfalls
- Ensure your server correctly supports HTTP/3 and QUIC.
- Regularly update your browser and server to the latest versions to benefit from performance improvements and security patches.
Troubleshooting and Debugging of WebTransport Protocol
Connectivity Issues
- Verify server support for WebTransport.
- Check network conditions and firewall settings.
Debugging Tips
- Use browser developer tools to monitor WebTransport activity.
- Log connection states and data transfers for easier debugging.
Best Practices
- Regularly test your implementation under different network conditions.
- Handle errors gracefully to maintain a robust connection.
Implementing WebTransport in Real-world Applications
Live Streaming
WebTransport can be used to deliver live video and audio streams with minimal latency, enhancing user experience in real-time applications.
Online Gaming
The protocol supports the rapid exchange of game state updates, crucial for maintaining synchronization in multiplayer games.
Collaborative Tools
WebTransport enables real-time collaboration by facilitating instant updates across users, making it ideal for applications like online whiteboards and document editors.
By implementing WebTransport in your projects, you can take advantage of its low-latency, secure, and efficient data transfer capabilities, providing a superior user experience in real-time applications.
Conclusion
The WebTransport protocol is a powerful tool for modern web development, offering low-latency, secure, and efficient bi-directional communication. By leveraging HTTP/3 and QUIC, WebTransport opens new possibilities for real-time applications, enhancing user experiences in fields like live streaming, online gaming, and collaborative tools. Start integrating WebTransport into your projects to harness its full potential.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ